Core concepts
Generic types
PHP autodoc supports generic types by analyzing class and function definitions for accurate type inference across generic collections, class strings, and extended classes.
Example 1
/**
* @template T
*/
class GenericClass
{
public function __construct(
/**
* @var T
*/
public $data,
) {}
}
public function genericExample1(): array
{
$animals = ['cat', 'dog', 'capybara'];
return [
'with_int_parameter' => new GenericClass(rand()),
'with_string_parameter' => new GenericClass('test'),
'with_array_parameter' => new GenericClass([
'elephant',
...$animals,
]),
];
}
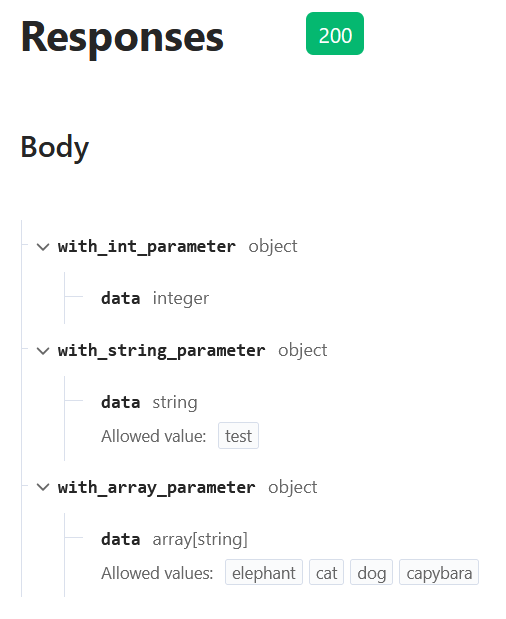
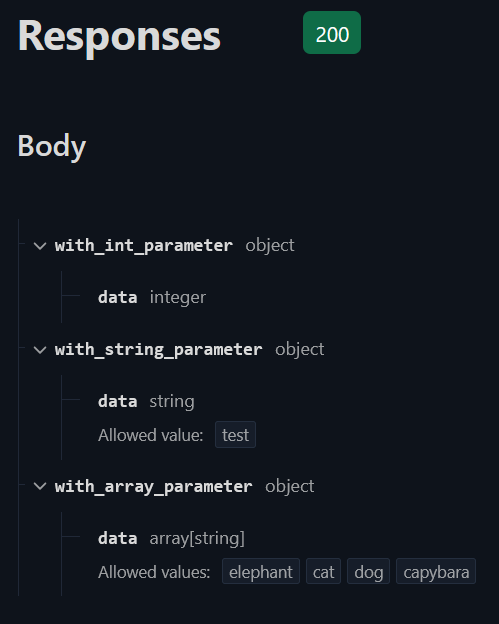
This example demonstrates how PHP autodoc supports generics in PHP classes and understands array destructuring to infer possible array values.
Note: Possible value detection for response types is disabled by default. To enable it, set
openapi.show_values_for_scalar_types
to true in your configuration file.
Example 2
This example demonstrates extension of generic class and how PHP autodoc remembers class-string variables to determine constructed instance type even when it is not specified using PHPDoc annotations.
/**
* @extends GenericClass<int>
*/
class ClassExtendingGenericClass extends GenericClass
{
public function __construct(
public int $n,
) {
parent::__construct($n);
}
}
public function genericExample2(): array
{
$integer = 1;
$date = new \DateTime();
return [
'generic' => $this->getInstanceWithPhpDoc(
className: GenericClass::class,
classConstructorParam: $date,
),
'generic_without_phpdoc' => $this->getInstance(
className: GenericClass::class,
classConstructorParam: $date,
),
'extended' => $this->getInstanceWithPhpDoc(
className: ClassExtendingGenericClass::class,
classConstructorParam: $integer,
),
'extended_without_phpdoc' => $this->getInstance(
className: ClassExtendingGenericClass::class,
classConstructorParam: $integer,
),
];
}
/**
* @template TClass of GenericClass
* @template TParam
*
* @param class-string<TClass> $className
* @param TParam $classConstructorParam
*
* @return TClass<TParam>
*/
private function getInstanceWithPhpDoc(
string $className,
mixed $classConstructorParam,
): object
{
// The return type is analyzed from PHPDoc comments
}
/**
* In some cases, when the class name is hard-coded, autodoc can
* determine what class is being constructed from variable.
*
* In this case the return type is analyzed from method body
* since `@return` tag is not specified.
*/
private function getInstance(
string $className,
mixed $classConstructorParam,
): mixed
{
return new $className($classConstructorParam);
}
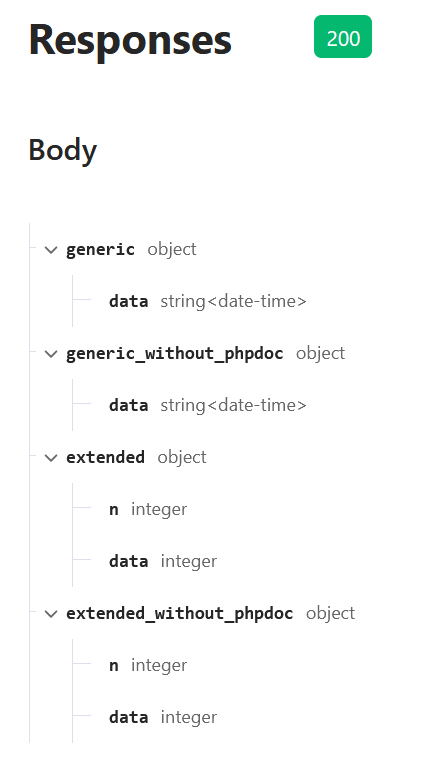
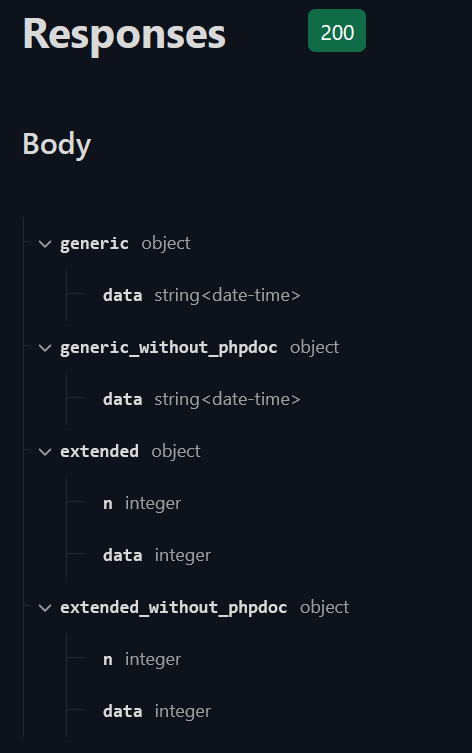